Tonight's Silverlight play involves Silverlight 4 Web Cam API, and SharePoint 2010 Client Object Model.
SILVERLIGHT 4 WEBCAM API
Silverlight 4's WebCam API is relatively simple:
_captureVideoDevice = CaptureDeviceConfiguration.GetDefaultVideoCaptureDevice();
_captureSource = new CaptureSource();
VideoBrush brush = new VideoBrush();
brush.SetSource(_captureSource);
WebCameraCapture.Fill = brush; // fill rectangle "render" area
var device = _captureVideoDevice.FriendlyName; // check device name during debug
if (CaptureDeviceConfiguration.AllowedDeviceAccess || CaptureDeviceConfiguration.RequestDeviceAccess())
{
_captureSource.Start();
}
The most interesting part is probably the RequestDeviceAccess call. This call must be initiated in an UI event (click) and will raise the following dialog
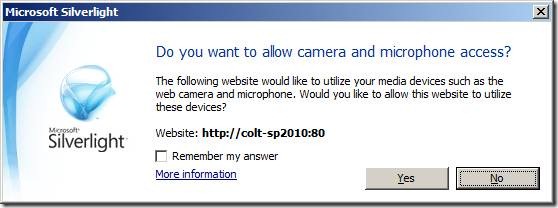
TAKING SNAPSHOTS
Once the camera is rolling, clicking the button takes a snapshot and saves it back to the document library.
WriteableBitmap bmp = new WriteableBitmap(WebCameraCapture, null);
MemoryStream stream = new MemoryStream();
// didn't want to save as bitmap - convert to JPEG first
EncodeJpeg(bmp, stream);
var fileCreationInfo = new FileCreationInformation();
fileCreationInfo.Content = stream.ToArray();
fileCreationInfo.Url = string.Format("pic_{0}.jpg", DateTime.Now.Ticks);
var _documentLibrary = _clientContext.Web.Lists.GetByTitle("ListName");
var uploadFile = _documentLibrary.RootFolder.Files.Add(fileCreationInfo);
_clientContext.Load(uploadFile);
_clientContext.ExecuteQueryAsync(delegate{}, delegate{});
In this screenshot I've added the Silverlight web part on top of the asset library list.
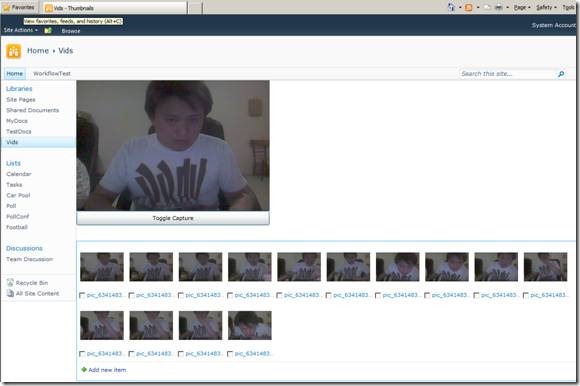
WHAT ABOUT VIDEO?
This is where we'll probably get stuck. Silverlight 4 has API to access camera, and we're able to save bitmap data from the camera, but Silverlight 4 lacks the client side encoding libraries capable of saving the bitmap stream back into any meaningful format such as MPEG.
Until Microsoft provides one, or some 3rd party writes one, saving video back to SharePoint document library is going to get put on hold.
REFERENCE
The code for converting to JPEG I copied from http://stackoverflow.com/questions/1139200/using-fjcore-to-encode-silverlight-writeablebitmap.
DOWNLOAD
The Silverlight Camera XAP binaries are in this XAP file
The Silverlight Camera Sandbox Solution and read me file
INSTALLATION
- Upload the XAP file to a document library, copy the URL to this XAP file.
- Create a document library to host all the pictures, open the web part zones on the view page and insert a Silverlight Web Part - use the URL to XAP from step 1.
- Remember to refresh the page to see new pictures - the list doesn't refresh automatically. You can switch on async properties in the web part editor for the pictures and it will update itself on a timer basis.
UPDATE
20 July - Added sandbox solution WSP and a simple read-me file